Useful utility views for every Django project
A small collection of simple utility views that I add to all my Django projects.
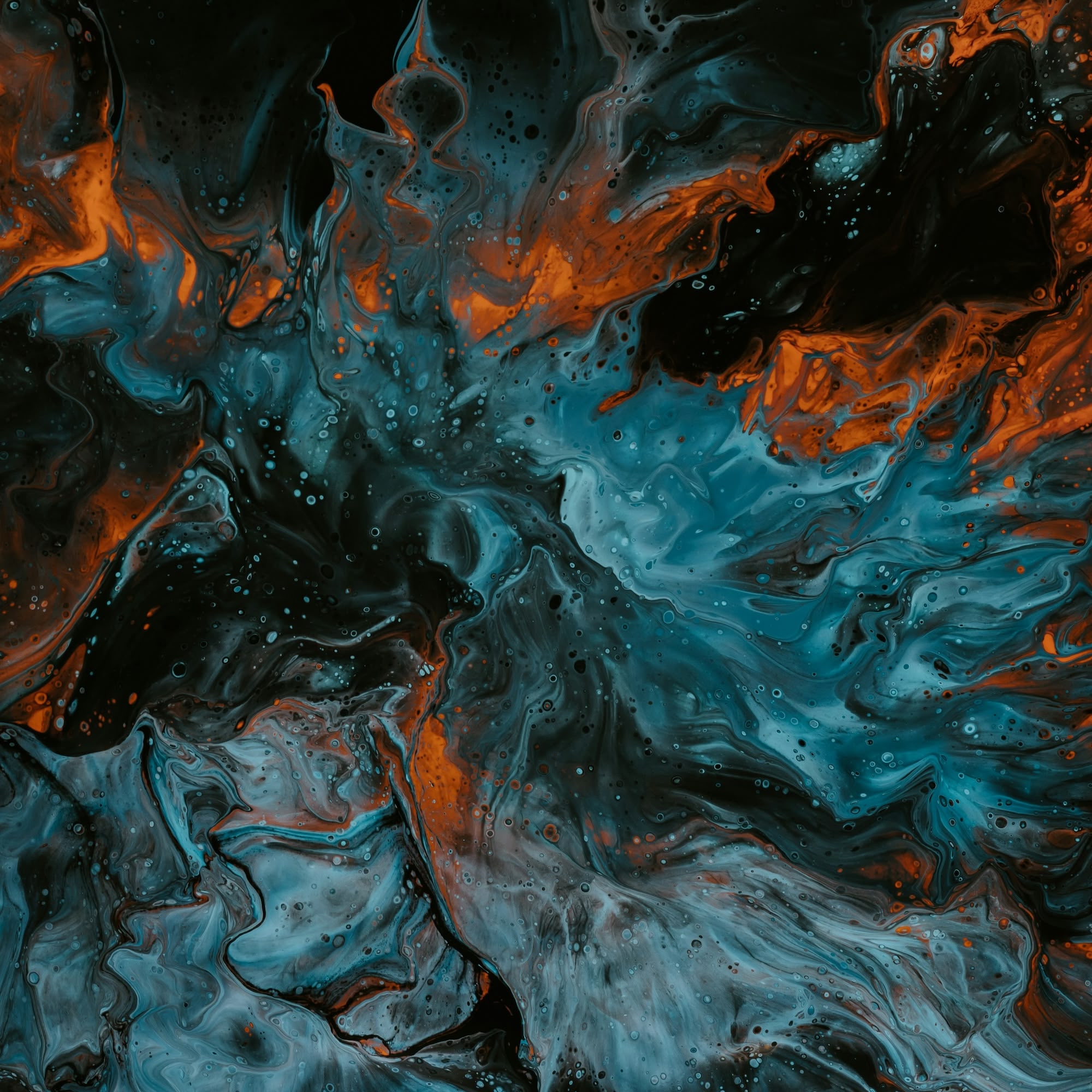
There are a few very simple utility views I drop into every Django project that help me when it comes to deploying my projects:
- A health-check endpoint
- An error-check endpoint
- A logging-check endpoint
- A timeout-check endpoint
These are invaluable when you launch your project on a platform and need to quickly validate that everything is working as it should.
Here are the views that I usually place in utils/views.py
:
import logging
import time
from django.http import HttpResponse
from django.views import View
logger = logging.getLogger(__name__)
class HealthCheckView(View):
def get(self, request, *args, **kwargs):
return HttpResponse("OK", content_type="text/plain")
class ErrorCheckView(View):
def get(self, request, *args, **kwargs):
raise Exception("Testing error handling")
class LoggingCheckView(View):
def get(self, request, *args, **kwargs):
logger.info("Responding to logging check request with a test log message")
return HttpResponse("OK", content_type="text/plain")
class TimeoutCheckView(View):
def get(self, request, *args, **kwargs):
time.sleep(45)
return HttpResponse("OK", content_type="text/plain")
and the accompanying urls.py
:
from utils.views import ErrorCheckView
from utils.views import HealthCheckView
from utils.views import LoggingCheckView
from utils.views import TimeoutCheckView
util_urlpatterns = [
path("health-check/", HealthCheckView.as_view(), name="health-check"),
path("logging-check/", LoggingCheckView.as_view(), name="logging-check"),
path("error-check/", ErrorCheckView.as_view(), name="error-check"),
path("timeout-check/", TimeoutCheckView.as_view(), name="timeout-check"),
]
url_patterns = [
path("", include(util_urlpatterns)),
]
Health Check
/health-check
This view simply returns an "OK" message. This is a useful endpoint for uptime-monitoring (I monitor all of my projects with UptimeRobot).
Having the same URL across all projects makes it easy to remember but also easy to filter out of logs. It's also useful on platforms like AWS where you need to add a health-check URL for autoscaling etc.
Logging Check
/logging-check
This view returns a test log message. This is useful when configuring and testing a 3rd party logging tool (I use BetterStack), in particular when you want to make sure your formatting is working correctly.
Error Check
/error-check
Throws a test exception. This is useful when configuring your error monitoring services (I use Sentry) to ensure that errors are being delivered correctly.
Timeout Check
/timeout-check
This delays the response for a number of seconds. This can be very useful for testing PAAS vendors where you're not able to manage the server configuration.
For example, I use Digital Ocean App Platform a lot, which has a gotcha that all requests timeout after 60 seconds. You have no control over this timeout so a utility view like this one is useful for testing and confirming the behaviour.
You obviously need to be careful exposing this view to the public. If someone finds it, they can easily clog up your app.
Have you got any other utility views that you add to every project? Let me know on Bluesky.